// Create Modifiers for each Button style
struct InfoButton: ViewModifier {
func body(content: Content) -> some View {
content
.padding(15)
.background(Color(UIColor.systemBlue).opacity(0.2))
.foregroundColor(Color(UIColor.systemBlue))
.cornerRadius(10)
}
}
struct SuccessButton: ViewModifier {
func body(content: Content) -> some View {
content
.padding(15)
.background(Color(UIColor.systemGreen).opacity(0.2))
.foregroundColor(Color(UIColor.systemGreen))
.cornerRadius(10)
}
}
struct WarningButton: ViewModifier {
func body(content: Content) -> some View {
content
.padding(15)
.background(Color(UIColor.systemOrange).opacity(0.2))
.foregroundColor(Color(UIColor.systemOrange))
.cornerRadius(10)
}
}
struct DangerButton: ViewModifier {
func body(content: Content) -> some View {
content
.padding(15)
.background(Color(UIColor.systemRed).opacity(0.2))
.foregroundColor(Color(UIColor.systemRed))
.cornerRadius(10)
}
}
// Extend button with custom function that simplify modifier assignment
extension Button {
func info() -> some View {
return self.modifier(InfoButton())
}
func success() -> some View {
return self.modifier(SuccessButton())
}
func warning() -> some View {
return self.modifier(WarningButton())
}
func danger() -> some View {
return self.modifier(DangerButton())
}
}
// How to use
struct ContentView: View {
var body: some View {
VStack(spacing: 10) {
Button(action: {}) {
Text("Info Button").frame(maxWidth: .infinity)
}.info()
Button(action: {}) {
Text("Success Button").frame(maxWidth: .infinity)
}.success()
Button(action: {}) {
Text("Warning Button").frame(maxWidth: .infinity)
}.warning()
Button(action: {}) {
Text("Danger Button").frame(maxWidth: .infinity)
}.danger()
}
}
}
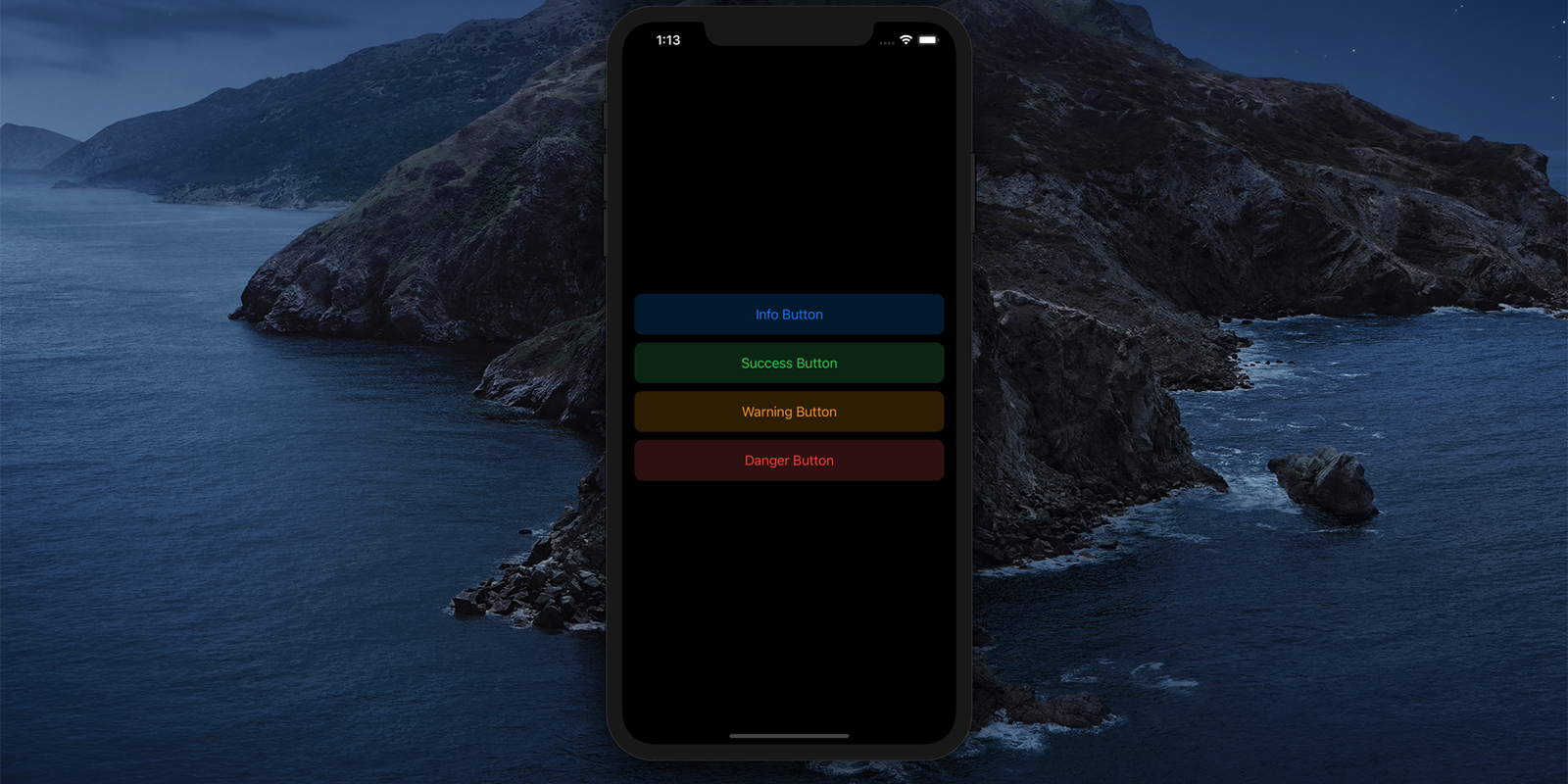
Button style modifiers
by Kane
This Component demonstrates how you can create custom modifiers for any SwiftUI view.
In this case - Button has been extended with 4 modifiers: info, success, warning, danger.
In this case - Button has been extended with 4 modifiers: info, success, warning, danger.